When an application is installed on an iOS device, it comes with its own allocated sandboxed space, consisting of various directories to store and manage files. One such directory, designated for temporary data, is known as the ‘tmp’ directory. This guide delves into the intricacies of handling files within this specific directory, covering operations such as creation, retrieval, and deletion of files. This tutorial is tailored for iOS 8.1, utilizing Xcode version 6.1.
Setting the Stage: Initializing Your Project
Crafting Your Application in Xcode:
- Initiating a New Project: Launch Xcode and opt to create a new ‘Single View Application’;
- Configuration;
- Product Name: Designate your project with the name ‘IOS8SwiftFileManagementTutorial’;
- Organizational Details: Fill in the ‘Organization Name’ and ‘Organization Identifier’ fields with values that resonate with your personal or company branding;
- Programming Language: Ensure that ‘Swift’ is selected as the programming language;
- Device Specification: Verify that the ‘Devices’ option is set exclusively to ‘iPhone’.
User Interface Design: Crafting the Visual Elements
Creating an Intuitive Layout with Storyboard:
- Button Integration: Within the Storyboard, incorporate four buttons onto the main view.
- Button Labeling: Assign distinct titles to each button:
- ‘Create File’;
- ‘List Directory’;
- ‘View File Content’;
- ‘Delete File’.
Auto Layout Configuration:
- Select all the buttons simultaneously using the ‘Ctrl’ key;
- Navigate to the ‘Resolve Auto Layout Issues’ tool, located at the bottom-right corner of the screen, and opt for ‘Add Missing Constraints’;
- This ensures that the buttons maintain their relative positioning regardless of device orientation or screen size.
Bridging UI and Logic: Connecting Buttons to Actions
Establishing Interactivity:
- Assistant Editor Utilization: Open the ‘Assistant Editor’ and ensure that ‘ViewController.swift’ is currently visible;
- Action Creation: For each button, perform a ‘Ctrl’ and drag operation to link them to the ‘ViewController’ class, subsequently creating individual actions.
Laying the Groundwork: Setting Up Properties
Defining Essential Components in ViewController.swift:
- Incorporate the following properties within the ‘ViewController’ class;
- var fileManager = NSFileManager(): This instance of ‘NSFileManager’ grants the capability to interact and modify the filesystem;
- var tmpDir = NSTemporaryDirectory(): A string representing the path to the temporary directory specific to the current user;
- let fileName = “sample.txt”: A constant holding the name of the file to be used throughout this tutorial.
Understanding the Components:
- NSFileManager: This class is instrumental for any file system related operations, providing methods to traverse, analyze, and modify contents within the filesystem;
- NSTemporaryDirectory: This function returns the path to the directory allocated for temporary files, aiding in storing data that is transient and can be purged when no longer needed;
- File Naming: The constant ‘fileName’ is initialized with the value “sample.txt”, serving as a placeholder for the file operations demonstrated in this tutorial.
Developing Helper Methods in Swift: Exploring enumerateDirectory
Understanding the Role of Helper Methods:
Before diving into the primary Action methods that drive the application’s functionality, it’s crucial to set the stage by introducing helper methods. One such invaluable assistant in this context is the enumerateDirectory function. This method stands as a pillar to determine the presence of specific files within a directory.
Breaking Down the enumerateDirectory Method:
Purpose: This function is designed to sift through the contents of a directory and discern the existence of a particular file.
Function Blueprint:
func enumerateDirectory() -> String? {
var error: NSError?
let directoryFiles = fileManager.contentsOfDirectoryAtPath(tmpDir, error: &error) as? [String]
if let files = directoryFiles {
if files.contains(fileName) {
println("sample.txt detected")
return fileName
} else {
println("File remains elusive")
return nil
}
}
return nil
}
Key Takeaways:
- The method fetches all file names inside the temporary directory and houses them in an array of String type;
- A subsequent check is performed to determine if our target file, sample.txt, exists within this array;
- A successful detection results in returning the file name, whereas a miss triggers a return of nil.
Crafting the createFile Action Method:
Objective: Create a new file within the temporary directory and populate it with a predetermined text content.
Method Design:
@IBAction func createFile(sender: AnyObject) {
let filePath = tmpDir.stringByAppendingPathComponent(fileName)
let fileContent = "Preliminary Text"
var error: NSError?
// Commence File Writing
if fileContent.writeToFile(filePath, atomically: true, encoding: NSUTF8StringEncoding, error: &error) == false {
if let errorMessage = error {
println("File creation stumbled")
println("\(errorMessage)")
}
} else {
println("File sample.txt carved in the tmp directory")
}
}
Core Components:
- File Path Construction: Stitch together the directory path with the file name to derive the complete file path;
- Content Determination: A predefined string, “Preliminary Text”, is set to be written into the file;
- File Writing Operation: Using the writeToFile method, the content is written to the specified file path;
- Success: A confirmation message is printed, indicating the successful creation of the sample.txt file in the temporary directory;
- Failure: In the unfortunate event of an error during the file creation process, a detailed error message is printed to aid debugging.
Recommendation: Always handle file-related errors gracefully. Incorporating robust error-handling mechanisms ensures that unexpected scenarios don’t disrupt the user experience.
Expanding on File Creation and Management in iOS Swift
Delving into File Writing and Handling Errors:
Upon the successful creation of the sample.txt file, complete with content, the application utilizes the writeToFile:atomically:encoding:error method to facilitate the writing of content to a file within the tmp directory. This operation, though seemingly straightforward, can encounter hiccups. For such cases, a well-crafted error message is displayed in the console, providing clear insights into what might have gone awry.
Implementing listDirectory: A Guide to Directory Enumeration
Objective: Navigate through the directory’s contents and bring them to light.
Action Method Blueprint:
@IBAction func listDirectory(sender: AnyObject) {
let filePresence = enumerateDirectory() ?? "Directory is Empty"
print("Directory Contents: \(filePresence)")
}
Decoding the Process:
- Calling enumerateDirectory: This helper function is invoked to scan the directory and check for the presence of files;
- Handling Absence of Files: Using the nil coalescing operator ??, the code gracefully handles scenarios where the directory might be devoid of files. In such cases, “Directory is Empty” serves as the fallback message;
- Console Output: Regardless of the outcome, the contents (or lack thereof) of the directory are printed to the console, ensuring transparency in the file enumeration process.
Tips for a Smooth Operation:
- Utilize descriptive messages and clear console outputs to maintain clarity in the debugging and development process;
- Ensure that error-handling and fallback mechanisms are in place to gracefully manage scenarios where files might be missing.
Crafting viewFileContent: Unveiling File Contents
Mission: Retrieve and display the contents of a specific file from the directory.
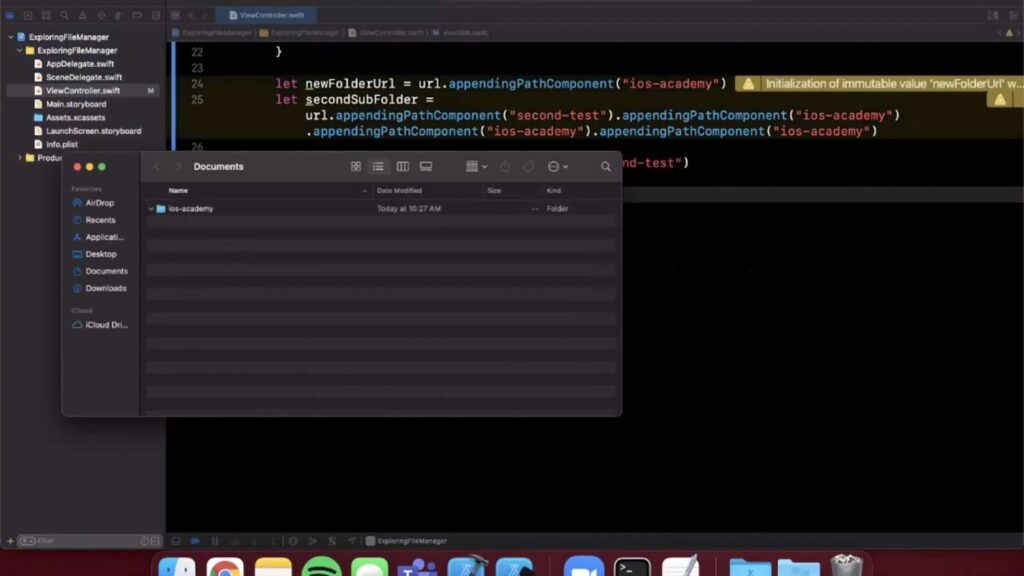
Method Design:
@IBAction func viewFileContent(sender: AnyObject) {
let filePresence = enumerateDirectory() ?? ""
let filePath = tmpDir.stringByAppendingPathComponent(filePresence)
let fileContents = NSString(contentsOfFile: filePath, encoding: NSUTF8StringEncoding, error: nil)
if let content = fileContents {
print("File Contents: \(content)")
} else {
print("File remains elusive")
}
}
Key Elements:
- Fetching File Path: The path to the file is constructed, paving the way to access its contents;
- Reading File Contents: Utilizing the contentsOfFile:encoding:error method, the content is retrieved and attempted to be cast to an NSString;
- Displaying Results: Depending on whether the file is present and readable, the content is either displayed, or a message indicating the file’s absence is printed;
- Pro Tip: Always ensure proper error handling when dealing with file reading operations to prevent unexpected crashes and enhance user experience.
Unraveling deleteFile: A Journey of File Deletion
Endgame: Remove a specific file from the directory, ensuring a clean slate.
Action Method Explained:
@IBAction func deleteFile(sender: AnyObject) {
var error: NSError?
if let filePresence = enumerateDirectory() {
let filePath = tmpDir.stringByAppendingPathComponent(filePresence)
fileManager.removeItemAtPath(filePath, error: &error)
} else {
print("File is Missing in Action")
}
}
Crucial Points:
- Locating the File: The enumerateDirectory function is once again employed to identify and locate the file in question;
- Initiating Deletion: Leveraging the removeItemAtPath:error method, an attempt is made to delete the file;
- Feedback: The console receives a message whether the file was successfully located and, if so, whether the deletion operation was successful.
Enhancing User Experience: Providing clear and concise feedback helps in maintaining transparency, aiding developers in troubleshooting and ensuring a smooth user interaction.
Bringing it All Together: Testing and Observations
The Final Act: With all the methods implemented, it’s time to build and run the project. Navigate through the user interface, interact with the buttons, and keep a keen eye on the console. This will be the stage where the results of each action, be it file creation, listing directory contents, viewing file content, or deleting a file, unfold before your eyes. Each button press correlates to a specific action, and the console plays a pivotal role in relaying the outcomes, ensuring a comprehensive understanding of the file management operations at hand.
Tips for Effective Testing:
- Test each functionality independently to ensure each component operates as expected;
- Pay close attention to the console output, as it provides invaluable insights and immediate feedback on the actions performed;
- In case of unexpected behavior, utilize breakpoints and debug tools to trace and rectify the issues.
By adhering to these guidelines, developers and users alike can navigate through the intricacies of file management within an iOS application, ensuring a robust and seamless experience. Also, learn how to create a basic yet functional calculator application!
Conclusion
In summary, this comprehensive guide has navigated through the intricacies of file management within an iOS application, using Swift as the programming vessel. From the initial stages of creating a well-structured environment for file operations, to implementing specific functionalities such as file creation, directory listing, content viewing, and file deletion, each aspect has been covered in depth, ensuring a holistic understanding and hands-on experience for developers.
Mastering file management in iOS is a critical skill for any Swift developer, and this guide serves as a stepping stone towards achieving that mastery. With the knowledge gained, developers are now well-prepared to handle files gracefully, ensuring a seamless and efficient user experience in their iOS applications.