Categories are a significant feature in Objective-C, enabling developers to extend classes without the need to modify their original implementation. This allows for adding functionalities to pre-existing classes in a clean and organized manner. Whether you’re just starting out with Objective-C or have extensive experience, this tutorial will provide a clear understanding of how to effectively use category extensions. Let’s get started and learn how to add and utilize categories in Objective-C.
Basic Concepts
Concept | Description |
---|---|
Objects | Objective-C is based on object-oriented programming, utilizing objects to represent and manage data. Objects are instances of classes, acting as blueprints for object creation. |
Messages | Instead of calling methods, Objective-C employs a messaging system. In this approach, you send messages to objects to invoke their behaviors, a fundamental aspect of its syntax and behavior. |
Interface and Implementation | Objective-C class definitions usually consist of two parts: the interface (defined in a .h file) outlining the class’s public interface and the implementation (in a .m file) containing the actual code that implements the class’s behavior. |
Objective-C Language Tutorial
Categories enable the extension of an object’s capabilities without the need for subclassing or modifying the original object. Typically, categories are employed to append methods to a pre-existing class. In this guide, we’ll introduce a category to the UISwitch class, allowing us to modify the switch’s colors.
Launch Xcode and initiate a new Single View Application. Label the product as “CategoryDemo” and proceed to populate the Organization Name, Company Identifier, and Class Prefix fields with your regular inputs. Ensure you’ve chosen only iPhone in the Devices section, deactivated the Use Storyboards option, and activated the Use Automatic Reference Counting option.
- To begin, we’ll set up our User Interface;
- Navigate to ViewController.xib and place a UISwitch onto the primary View;
- Your main View should appear as described;
- Activate the assistant editor and access ViewController.m;
- Use Ctrl+drag from the switch towards the interface segment to generate an IBOutlet property;
- Next, choose the New -> File alternative and pick the “Objective-C category” blueprint;
- Label the category “CustomSwitch” and select UISwitch as the associated class;
- This action produces two files: UISwitch+CustomSwitch.h and UISwitch+CustomSwitch.m;
- Proceed to UISwitch+CustomSwitch.h to input a method declaration.
@interface UISwitch (CustomSwitch)
+ (id)UISwitchWithTintColor:(UIColor *)tintColor onTintColor:(UIColor *)onTintColor thumbTintColor:(UIColor *)thumbTintColor;
@end
Next, navigate to UISwitch+CustomSwitch.m and execute the UISwitchWithTintColor:onTintColor:thumbTintColor method.
+ (id)UISwitchWithTintColor:(UIColor *)tintColor onTintColor:(UIColor *)onTintColor thumbTintColor:(UIColor *)thumbTintColor
{
[[UISwitch appearance] setOnTintColor:onTintColor];
[[UISwitch appearance] setTintColor:tintColor];
[[UISwitch appearance] setThumbTintColor:thumbTintColor];
return self;
}
In this step, we’re just assigning colors to the UISwitch. Navigate to the ViewController.m file and incorporate our Category Header file.
#import "UISwitch+CustomSwitch.h"
Change the viewDidLoad method.
- (void)viewDidLoad
{
[super viewDidLoad];
self.mySwitch = [UISwitch UISwitchWithTintColor:[UIColor redColor] onTintColor:[UIColor blueColor] thumbTintColor:[UIColor greenColor]];
}
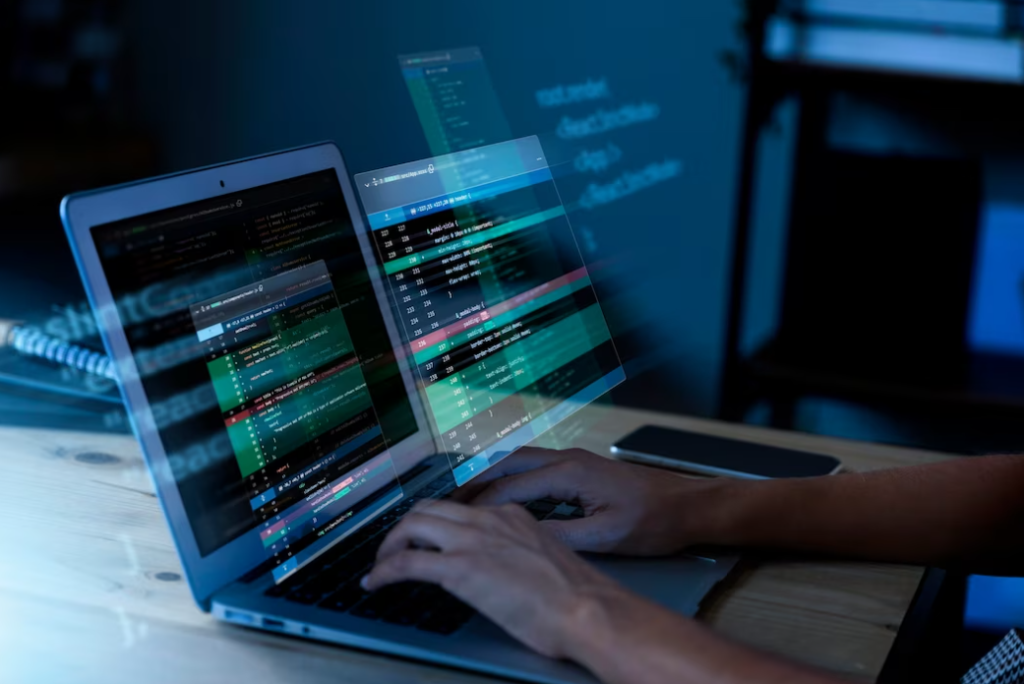
We invoke the category method to tailor the UISwitch colors. Compile and execute, then toggle the switch to view the personalized shades.
The CategoryDemo source code is accessible for download from the ioscreator repository on GitHub.
Conclusion
While Swift has largely overtaken Objective-C in terms of popularity for new projects, Objective-C remains a critical language for many existing projects and legacy systems. Understanding Objective-C is essential for any developer working in the Apple ecosystem. This tutorial has provided a brief overview, but there’s much more to learn as you delve deeper into the language and its rich set of frameworks and tools.
Understanding Objective-C is crucial for Apple developers. After exploring its frameworks and tools, you might also appreciate our guide on how to use SwiftUI’s color picker.