Web Views act as a bridge between native app functionalities and web content, enabling users to view online resources without ever leaving an app. For iOS developers, this means crafting applications that seamlessly integrate web-based content, be it for user guides, news feeds, or other external sources.
Why Are Web Views Important in iOS Development?
- User Experience: Web Views mean users won’t have to jump between the app and a web browser, providing a smooth UX;
- Content Versatility: Easily update web content without updating the app;
- Feature Integration: Combine native app functionalities with web-based resources.
Did you know? Apple introduced the WebKit framework in iOS 2.0, paving the way for developers to embed web content directly in their apps.
Setting Up Your Development Environment
Before diving in, let’s ensure you’ve got all the tools necessary:
- Xcode – The official development environment for iOS;
- A Mac computer running the latest macOS;
- An active Apple Developer account.
WebKit: The Heart of iOS Web Views
WebKit, Apple’s open-source web browser engine, powers web views in iOS. It provides a range of classes to embed web content.
- WKWebView: The modern way to embed web content, replacing the deprecated UIWebView;
- WKNavigation: Manages a webpage’s navigation process.
Crafting Your First Web View with WKWebView
Here’s a quick guide:
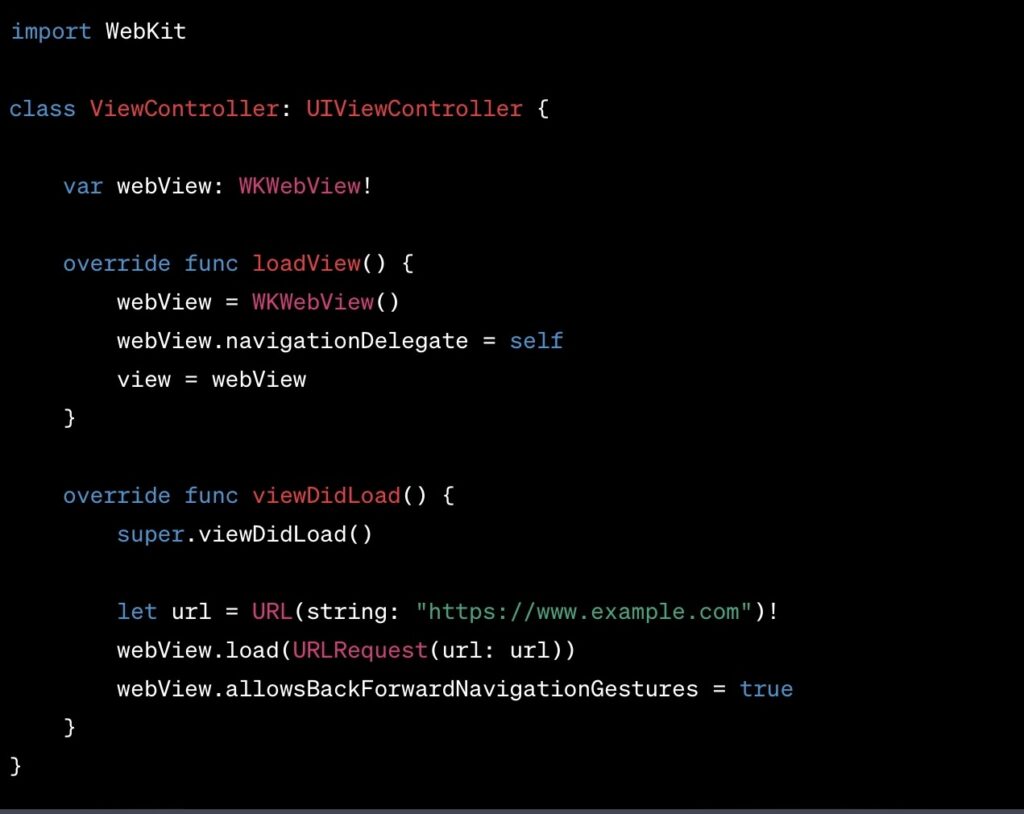
This code snippet ensures you’ve created a WebView and directed it to a specific URL.
Enhancing User Experience with WKNavigation
Consider a scenario where you want to show a loading spinner every time a webpage loads. WKNavigation makes this easy:
- Detect when the page starts to load;
- Show a spinner;
- Hide the spinner once the page has loaded.
Handling Cookies and Sessions
Web Views not only display content but can also handle cookies and sessions. This is paramount for web pages requiring user authentication.
Dealing with JavaScript in WKWebView
WKWebView supports executing JavaScript. This ability expands the range of tasks an iOS app can perform when interacting with web content.
For instance, to retrieve the title of a webpage:
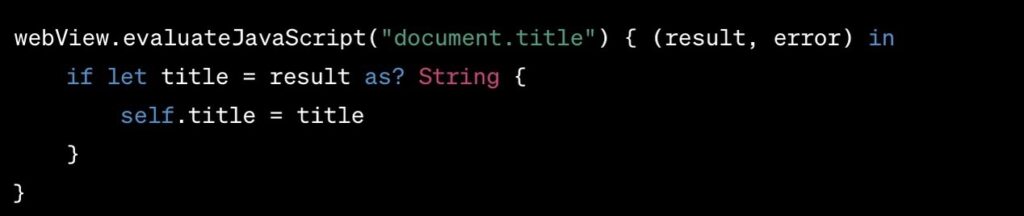
Debugging and Performance Monitoring
Like with any other component, monitoring the performance and ensuring a bug-free experience is paramount. Utilize tools like Safari’s Web Inspector to dive deep into the WebView content.
Best Practices for Integrating Web Views
- Avoid Overusing: Remember, Web Views can impact app performance;
- Stay Updated: Apple frequently updates WebKit; ensure your implementations are up-to-date;
- Security: Always validate URLs and web content to keep user data safe.
Imagine embedding web views as placing a window in a room. While the window offers a view outside, it shouldn’t compromise the room’s comfort or safety.
Conclusion
Web Views in iOS open up a world of possibilities for developers. By integrating web content seamlessly, you can craft richer and more versatile apps. However, like any tool, it’s essential to use them wisely, focusing on user experience, performance, and security.
Frequently Asked Questions
WKWebView is the modern approach, offering more features and better performance. UIWebView is now deprecated.
Absolutely! WKWebView supports the execution of JavaScript, adding more flexibility to your app.
Web Views can manage cookies and sessions, crucial for web pages requiring user login or personalization.
While Web Views are generally safe, always validate URLs and web content to ensure user data security.
Regularly! Apple frequently releases updates, so stay abreast to provide the best user experience.